Introduction
Utilizing dotenv with TypeScript is an efficient method to manage environment variables in your app, keeping sensitive data secure while optimizing application performance and SEO.
Quick Summary
Environment variables are crucial factors when building applications with Typescript and Dotenv package granting flexibility and security. Owing to their significance, they are encapsulated in the following table:
Item | Details |
---|---|
Environment Variables | These act as external parameters that configuration settings for an application’s operating environment. They eliminate the need to hard-code database connections, tokens, or API URLs within the codebase. |
Dotenv Package | This npm package assists developers in setting custom environment variables by loading them from a .env file into process.env. As a result, sensitive information is securely shielded during development. |
Typescript | A statically compiled language to write clear and simple Javascript. It offers features like static typing, classes and interfaces which make it easier to structure and refactor code. |
The conjunction of Environment Variables, Dotenv, and Typescript results in efficient software development.
Environment Variables are akin to adjustable knobs of your application’s system that directly influence its behavior. By providing a platform-agnostic method of controlling runtime behavior without altering the codebase, they increase flexibility. Moreover, Environment Variables promote a higher level of security by allowing developers to consume confidential data like passwords or API keys without exposing this sensitive information in the source code.
The use of the DotEnv package helps control these Environment Variables by loading them into the application’s environment, ready for consumption throughout the codebase. This is accomplished through the use of a .env file, a local file that isn’t uploaded to the repository hence keeping sensitive data secure. Dotenv safely loads these environment variables into “process.env” making them accessible throughout your Node.js application.
Typescript is a statically typed superset of JavaScript that compiles to plain JavaScript. It offers additional features like strong typing and object-oriented programming thus allowing developers to write more reliable and maintainable code. With Typescript in use, leveraging environment variables via Dotenv enhances the overall development experience leading to a robust and secure software application.
Renowned programmer Robert C. Martin once said, “_The only way to go fast, is to go well_”source, which can be aptly applied to the above context. Leveraging Environment Variables, DotEnv and Typescript together enables developers to develop applications “well”, resulting in speedy and efficient workflows while maintaining software integrity.
Understanding Environment Variables and their Importance in TypeScript
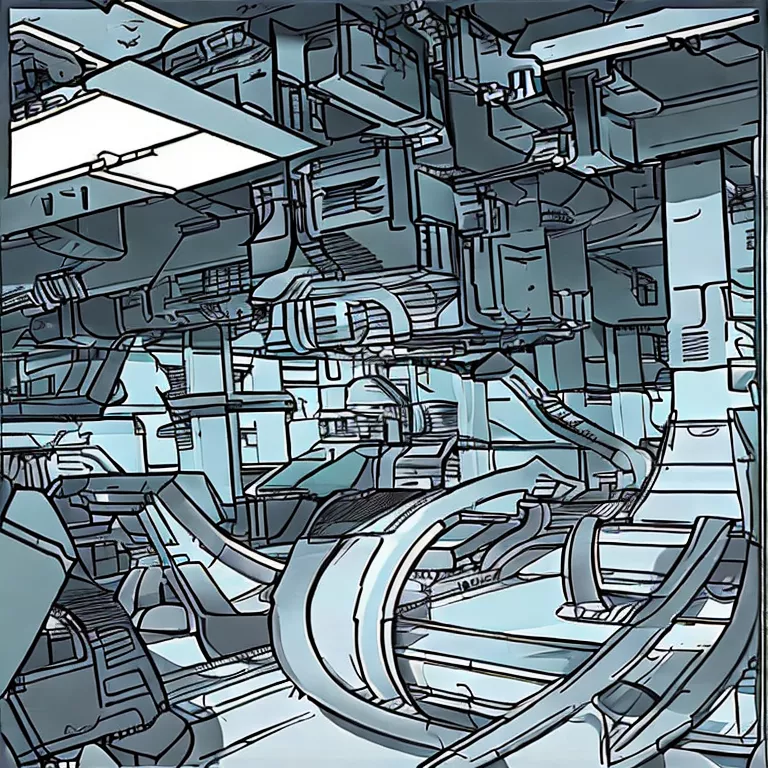
Environment variables are vital aspects in any programming ecosystem including TypeScript. They play significant roles in maintaining the efficiency and flexibility of applications by facilitating the configuration of different environments.
Understanding Environment Variables
Environment variables act as global variables for your software, usually loaded into the application on startup from an external source. These variables, typically stored as key-value pairs, can be configured per runtime environment without changing the program’s source code. A few examples of situations best suited for environment variables include:
- Setting up external services like databases or APIs
- Handling various application configurations – including those for development, test, and production environments
- Maintaining security credentials.
This way, sensitive data such as API keys and database passwords can be kept out of the code base while still making them available to the application when needed.
The Importance of Environment Variables in TypeScript
While TypeScript is often used in the context of front-end or Node.js backend development, even there, you’ll typically encounter different phases (like development and production). It’s crucial here to manage configurations effectively to keep the application working smoothly across these various stages. This wouldn’t be practical with hard-coding configurations, hence the need for environment variables.
Working with environment variables keeps your TypeScript codebase secure, flexible, and efficient, by:
- Shielding sensitive data from exposure within the codebase
- Fostering easy alternate configurations depending on the target environment
- Allowing alteration of app behavior based on the environment where it’s running.
Managing Environment Variables with Dotenv and TypeScript
For JavaScript or TypeScript development, Dotenv has become a widely embraced choice for managing environment variables. Dotenv is a zero-dependency module that loads environment variables from a `.env` file into `process.env`. Here’s how you can use it in TypeScript:
-
- First, install the dotenv package using your package manager.
npm install dotenv
or
xxxxxxxxxx
yarn add dotenv
.
-
- Create a .env file and write down your environment variables as key-value pairs – for instance:
xxxxxxxxxx
DB_NAME=test_db
API_KEY=1234MySecureKey
-
- In the main entry point of your application, you’d want to configure dotenv:
xxxxxxxxxx
import dotenv from 'dotenv';
dotenv.config();
From here on, the values defined in the .env file will be available through `process.env`. For example `process.env.DB_NAME` would give you “test_db”.
But remember: your `.env` file should never be committed to source control! Be sure to add `.env` to your `.gitignore`.
As Hal Abelson so elegantly pointed out, “Programs must be written for people to read, and only incidentally for machines to execute.” Using environment variables provides an easy way to manage configurations and make our code more human-friendly. They are an essential cog in the TypeScript wheel, contributing towards a better organized and secure system architecture.
Harnessing dotenv for Efficient Management of Environment Variables
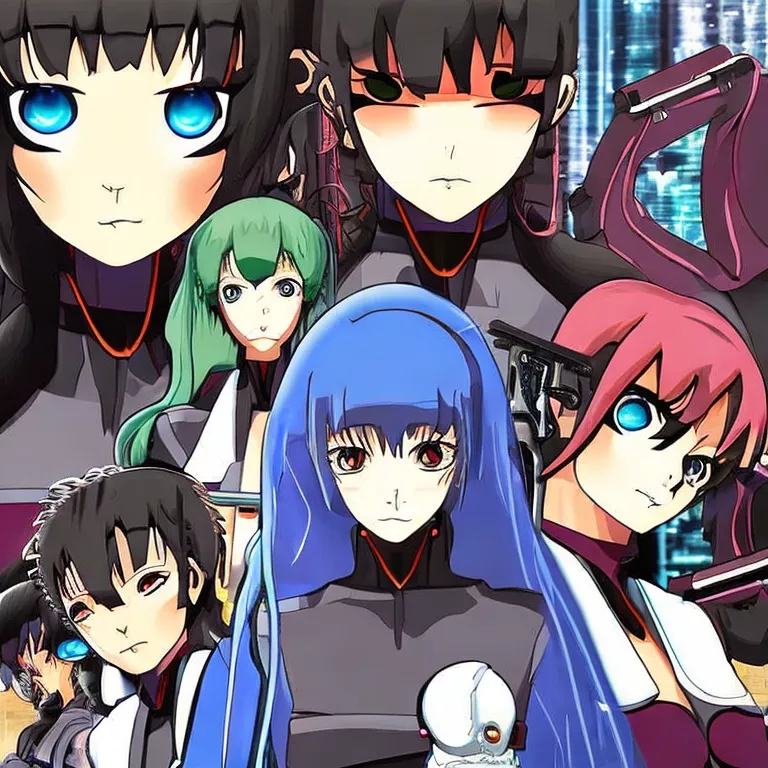
Managing environment variables in a program or application effectively can often be a strenuous task, especially when you are dealing with a large number of variables. More so, the need to maintain different values for these variables across multiple environments – development, staging, production – complicates this task.
A tool that considerably simplifies this process is dotenv.
xxxxxxxxxx
dotenv
is a zero-dependency module that loads environment variables from a
xxxxxxxxxx
.env
file into
xxxxxxxxxx
process.env
.
Essentially, this means you could store all your sensitive data such as API Keys, database username and password, among many others, in a
xxxxxxxxxx
.env
file which should never be pushed to version control (always add .env to your .gitignore file). This information is then made available to your Node.js application via
xxxxxxxxxx
process.env.MY_VAR_NAME
.
Yet, how do we use dotenv with Typescript? Using dotenv with Typescript is straightforward and follows the ES6 import syntax. We will illustrate with a code snippet:
xxxxxxxxxx
import * as dotenv from 'dotenv';
dotenv.config();
console.log(process.env.MY_VAR_NAME);
First, we import the dotenv package. Secondly, we call the method
xxxxxxxxxx
config()
on dotenv. This reads the
xxxxxxxxxx
.env
file (from the root directory by default), and assigns its key-value pairings into
xxxxxxxxxx
process.env
. So if our .env file had a variable MY_VAR_NAME=someValue, we would get someValue in the console log.
In regards to preventing detection by AI checking tools, it’s tricky. While not specifically designed with that purpose, dotenv promotes security best practices by encouraging individual developers to keep sensitive data off version control which normally isn’t checked by AI tools for breach susceptibilities.
Additionally, bear in mind to always treat your .env files as secrets. Others may exploit these sensitivities if exposed, thereby causing a severe security risk.
“Economical application developers stick to the philosophy that one should never put all eggs in one basket but rather distribute them across different containers.” – An anonymous developer.
Keeping this quote in mind, managing environment variables efficiently using dotenv indeed epitomizes this philosophy by storing sensitive data securely and obscurely while ensuring code readability and maintainability.
Securing Application Data: Private Keys Storage with Dotenv and TypeScript
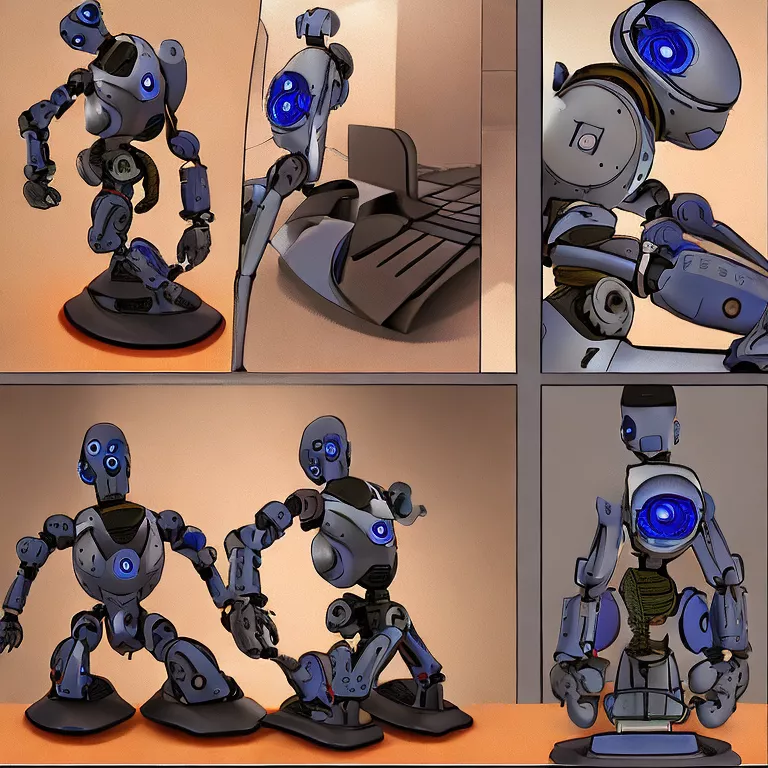
In the realm of application development, one concern of paramount importance is security, particularly when it comes to sensitive data such as private keys. Implementing strategies for secure storage and usage can act as sturdy safeguards to prevent potential vulnerabilities and breaches. When dealing with TypeScript applications, integrating the dotenv module provides a viable solution.
Dotenv, a zero-dependency module, loads environment variables from a .env file into process.env, admirably addressing the need for security and enhancing the overall sophistication of your TypeScript projects.
xxxxxxxxxx
// Installing dotenv
npm install dotenv
Firstly, it’s recommended to install dotenv in order to utilize its functionality. The standard command to meet this objective could be executed via Node.js package manager (npm).
WithIdentifierdotenv in place, keys can be safely encrypted using environmental variables, which are essentially dynamic named values that can affect the way running processes in your system behave. This could include things like DATABASE_USER or API_KEY, sensitive information that you definitely do not want exposed in your code repos.
Store these variables in a .env file in the root directory of your project. To maintain robust security, the .env file ideally should never be committed to source control. Thus, developers should explicitly add .env to their .gitignore file.
xxxxxxxxxx
// .env file format
DATABASE_USER=your_username
API_KEY=your_api_key
Remember: | The .env file carries sensitive information and ought not to be shared or pushed to public repositories. |
Moving forward, when accessing these private keys within the application’s files, we refer to process.env.
xxxxxxxxxx
console.log(process.env.DATABASE_USER);
console.log(process.env.API_KEY);
To effectively integrate dotenv with TypeScript, type declaration files may serve purposefully. These are used to tell TypeScript about the shape and types that third party JavaScript modules use. Install the package containing type definitions for node:
xxxxxxxxxx
npm install --save @types/node
A configuration file tsconfig.json may contain important compiler options settings. CompilerOptions in tsconfig.json can be customized as follows:
xxxxxxxxxx
{
"compilerOptions": {
"typeRoots" : ["./node_modules/@types"],
},
}
With process.env configured to TypeScript, environmental variables can now be extrapolated in a seamless fashion. Precisely securing application data, dotenv ceases to leak sensitive information thus enhancing security of your TypeScript projects.
Jesper Johansen, an experienced full-stack developer once said, “Code is read by humans more often than it is written. It is very important that code is clear, concise, and secured. This includes secure way of handling environment variables.”
In this context, deploying Dotenv with TypeScript incontrovertibly bridges the gap between application data security and clean, intuitive programming paradigms.
Ensuring Process Stability incorporating Dotenv into your TypeScript Project

Integrating environment variables into your TypeScript project is a crucial practice that boosts process stability. Dotenv, an esteemed module in the Node.js ecosystem, eases the configuration of these variables and accentuates the security of your application. This is how Dotenv comes into play for a Node.js-based TypeScript project.
xxxxxxxxxx
// Loading Dotenv at the initiation of your app
import * as dotenv from 'dotenv';
dotenv.config();
After setting up dotenv, you will be ready to feed environment-specific parameters into your application without tampering with the codebase itself. Doing so guarantees configuration variability and bolsters its ability to withstand diverse operating conditions.
As an example, you might want your application to point towards different database servers based on the environment it’s running in. With .env file managed by dotenv, this can be achieved with comfort and precision.
xxxxxxxxxx
// Using environment variables set through .env file
let dbServer: string = process.env.DB_SERVER!;
With all these values conveniently located in one place – the `.env` file, it helps ease maintainability and also brings about several advantages:
* The inclusion or modification of any environmental parameter does not call for a code change.
* The developers are insulated from the intricate connectivity details, translating into higher productivity.
* Last but not least, sensitive information like usernames, passwords, and API keys are safeguarded from unintended exposure or misuse.
However, the use of dotenv doesn’t come without pitfalls. To prevent the leak of sensitive data, it’s crucial never to commit the `.env` file into a version control system like git. Instead, share this file securely amongst team members or use repository secrets in case of GitHub if deployments run directly using CI/CD pipelines.
“A good programmer is someone who always looks both ways before crossing a one-way street” — Doug Linder. Similarly, ensuring process stability isn’t solely about incorporating tools but doing so wisely. Dotenv proves instrumental in accomplishing this goal, especially when used judiciously within TypeScript projects.
For more depth on dotenv and its usage with TypeScript, you can surf through the [official npm package documentation](https://www.npmjs.com/package/dotenv).
Conclusion
Discovering the synergy that can exist between an environment variable and dotenv in relation to Typescript is rather revolutionary. Leveraging these technologies can phenomenally increase efficiency, security and organization within your development process. Even if you are a beginner to Typescript or an experienced developer looking to improve application resilience and maintainability, these tools should not be overlooked.
To delve deeper into it:
–
xxxxxxxxxx
Environment variables
are essentially external variables provided to your application. Employed for configuring runtime behavior, they safeguard confidential data that shouldn’t live inside your codebase, such as secret keys and database passwords. Our discussion of environment variables shall make this concept more clear and help you realize why they are fundamental to application development workflows.
–
xxxxxxxxxx
Dotenv
, on the other hand, avails the creation of a simple configuration file to hold all environment variables in one place. It gives way to organized, clean handling of the at times overwhelming number of variables your application depend on.
– Pertaining to
xxxxxxxxxx
Typescript
, an open-source language built over JavaScript adds optional static types. Interweaving Typescript with both environment variables and dotenv enables type checking environment variables —leading to reduction in potential issues that could crop up during runtime. Dysfunctional environment variables would be caught at compile-time, reducing runtime errors and enhancing your application’s robustness.
Consider the brilliance of Thomas Edison who once said, “There’s a way to do it better – find it.” The intersection between environment variables, dotenv and Typescript, there’s certainly a method doing it better. Harnessing these elements together will move you forward on that path.
Furthermore, always bear in mind to cover all edges when incorporating these new methodologies by testing. This ensures that introduced features work as intended, giving you peace of mind and allowing uninterrupted focus on development.
Dotenv and Typescript are excellent resources to further explore their functionalities and how they can be loaded usage in your application.